SQL优化
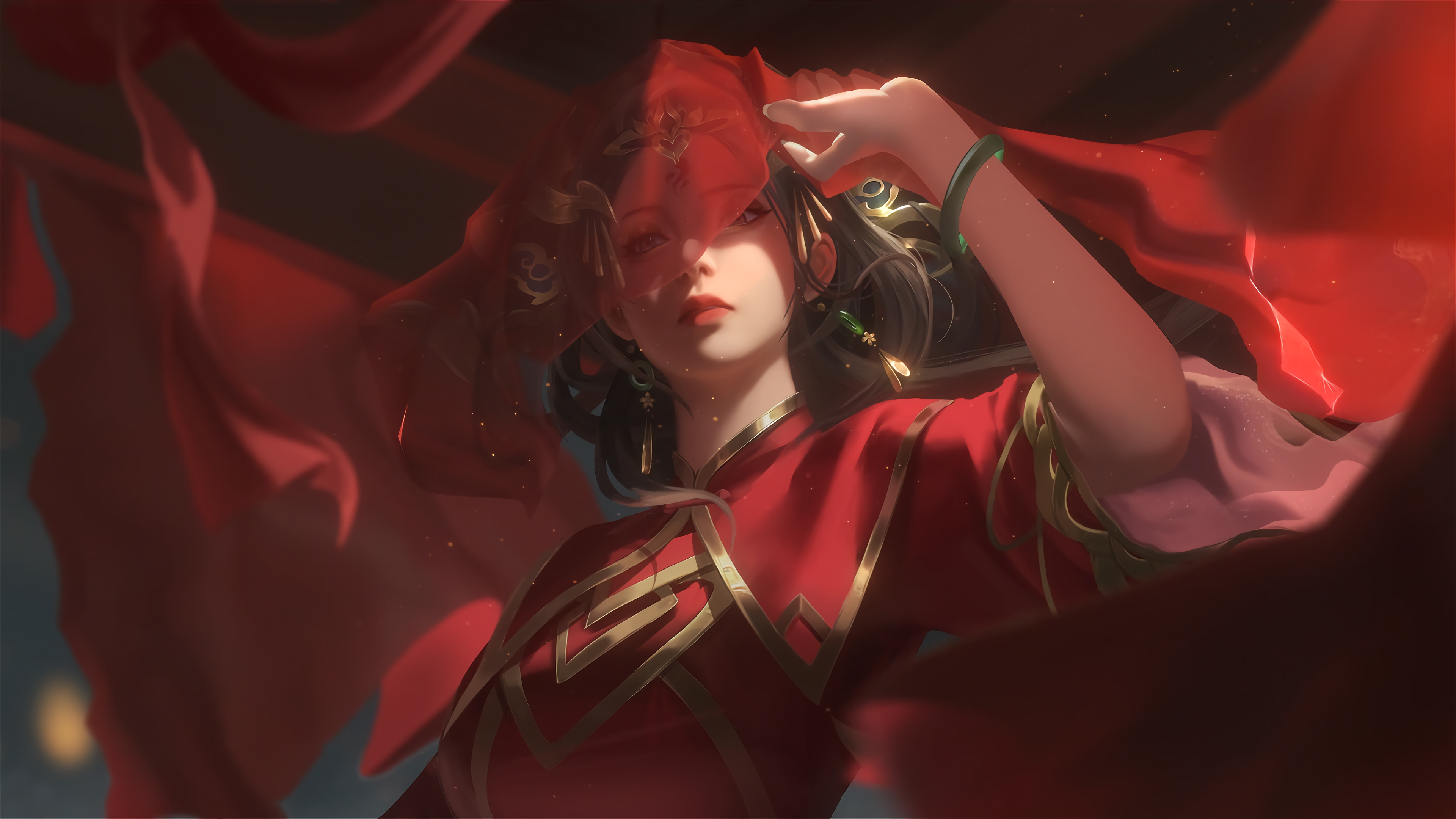
SQL优化
SerMs插入数据
批量插入数据
1 | Insert into tb_test values(1,'Tom'),(2,'Cat'),(3,'Jerry'); |
手动控制事务
1 | start transaction; |
主键顺序插入,性能要高于乱序插入
1 | 主键乱序插入 : 8 1 9 21 88 2 4 15 89 5 7 3 |
大批量插入数据
如果一次性需要插入大批量数据(比如: 几百万的记录),使用insert语句插入性能较低,此时可以使 用MySQL数据库提供的load指令进行插入。操作如下:
1 | -- 客户端连接服务端时,加上参数 -–local-infile |
order by优化
MySQL的排序有两种方式:
- Using filesort : 通过表的索引或全表扫描,读取满足条件的数据行,然后在排序缓冲区sort buffer中完成排序操作,所有不是通过索引直接返回排序结果的排序都叫 FileSort 排序。
- Using index : 通过有序索引顺序扫描直接返回有序数据,这种情况即为 using index,不需要 额外排序,操作效率高。
对于以上的两种排序方式,Using index的性能高,而Using filesort的性能低,我们在优化排序 操作时,尽量要优化为 Using index。
执行SQL
1 | explain select id,age,phone from tb_user order by age ; |
由于 age, phone 都没有索引,所以此时再排序时,出现Using filesort, 排序性能较低。
1 | -- 创建索引 |
建立索引之后,再次进行排序查询,就由原来的Using filesort, 变为了 Using index,性能 就是比较高的了。
创建索引后,根据age, phone进行降序排序
1 | explain select id,age,phone from tb_user order by age desc , phone desc ; |
也出现 Using index, 但是此时Extra中出现了 Backward index scan,这个代表反向扫描索 引,因为在MySQL中我们创建的索引,默认索引的叶子节点是从小到大排序的,而此时我们查询排序 时,是从大到小,所以,在扫描时,就是反向扫描,就会出现 Backward index scan。 在 MySQL8版本中,支持降序索引,我们也可以创建降序索引。
根据phone,age进行升序排序,phone在前,age在后。
1 | explain select id,age,phone from tb_user order by phone , age; |
排序时,也需要满足最左前缀法则,否则也会出现 filesort。因为在创建索引的时候, age是第一个 字段,phone是第二个字段,所以排序时,也就该按照这个顺序来,否则就会出现 Using filesort。
根据age, phone进行降序一个升序,一个降序
1 | explain select id,age,phone from tb_user order by age asc , phone desc ; |
因为创建索引时,如果未指定顺序,默认都是按照升序排序的,而查询时,一个升序,一个降序,此时 就会出现Using filesort。
为了解决上述的问题,我们可以创建一个索引,这个联合索引中 age 升序排序,phone 倒序排序。
创建联合索引(age 升序排序,phone 倒序排序)
1 | create index idx_user_age_phone_ad on tb_user(age asc ,phone desc); |
order by优化原则:
- 根据排序字段建立合适的索引,多字段排序时,也遵循最左前缀法则。
- 尽量使用覆盖索引。
- 多字段排序, 一个升序一个降序,此时需要注意联合索引在创建时的规则(ASC/DESC)。
- 如果不可避免的出现filesort,大数据量排序时,可以适当增大排序缓冲区大小 sort_buffer_size(默认256k)。
group by优化
在没有索引的情况下,执行如下SQL,查询执行计划:
1 | explain select profession , count(*) from tb_user group by profession ; |
然后,我们在针对于 profession , age, status 创建一个联合索引。
1 | create index idx_user_pro_age_sta on tb_user(profession , age , status); |
紧接着,再执行前面相同的SQL查看执行计划。
1 | explain select profession , count(*) from tb_user group by profession ; |
我们发现,如果仅仅根据age分组,就会出现 Using temporary ;
而如果是 根据 profession,age两个字段同时分组,则不会出现 Using temporary。原因是因为对于分组操作, 在联合索引中,也是符合最左前缀法则的
所以,在分组操作中,我们需要通过以下两点进行优化,以提升性能:
- 在分组操作时,可以通过索引来提高效率。
- 分组操作时,索引的使用也是满足最左前缀法则的。
limit优化
在数据量比较大时,如果进行limit分页查询,在查询时,越往后,分页查询效率越低。
优化思路: 一般分页查询时,通过创建 覆盖索引 能够比较好地提高性能,可以通过覆盖索引加子查 询形式进行优化。